Class-transformer
Deserialization is the opposite of serialization, in other words, deserialization is a process of creating an object from a stream of bytes. In the serialization process, the fields or properties of an object, the name of the class and the assembly that contains the class are converted to a stream of bytes.
- You can use JSON deserialization extension - cz.habarta.typescript.generator.ext.JsonDeserializationExtension - which generates methods that allow to 'deserialize' JSON data into instances of generated classes.It adds 'copy' methods to classes which receive pure object, create new instance of the class and recursivelly copy properties from data object to the instance of class.
- I started working with TypeScript about two years ago. Most of the time I read a JSON object from a remote REST server. This JSON object has all the properties of a TypeScript class. There is a question always buzz my head: How do I cast/parse the received JSON object to an instance of a corresponding class?
typestack/class-transformer: Decorator-based , class-transformer. Build Status codecov npm version. Its ES6 and Typescript era. Nowadays you are working with classes and constructor objects more then Class-transformer allows you to transform plain object to some instance of class and versa. Also it allows to serialize / deserialize object based on criteria. This tool is super useful on both frontend and backend.
class-transformer, Proper decorator-based transformation / serialization / deserialization of plain javascript objects to class constructors. class-transformer. Its ES6 and Typescript era. Nowadays you are working with classes and constructor objects more then ever. Class-transformer allows you to transform plain object to some instance of class and versa. Also it allows to serialize / deserialize object based on criteria. This tool is super useful on both frontend and backend.
Serialization, In doing so, it can apply rules expressed by class-transformer decorators on an entity/DTO class, as described below. Exclude properties#. Let's assume that we Beast Machines continued Beast Wars' size classes, but increased the price of Basic to US$7.. Supreme was introduced as a US$40 size class.Cheetor was the only Supreme in this line; a Supreme Optimus Primal toy was planned to be in this line, but was moved to Robots in Disguise.
Jsonnode
JsonNode (jackson-databind 2.7.0 API), Method for finding a JSON Object field with specified name in this node or its child nodes, and returning value it has. List<JsonNode>, findValues(String fieldName). The Jackson JsonNode class is the Jackson tree object model for JSON. Jackson can read JSON into an object graph (tree) of JsonNode objects. Jackson can also write a JsonNode tree to JSON. This Jackson JsonNode tutorial explains how to work with the Jackson JsonNode class and its mutable subclass ObjectNode.
Jackson JsonNode, We'll use JsonNode for various conversions as well as adding, modifying and removing nodes. 2. Creating a Node. public abstract JsonNode findParent(String fieldName) Method for finding a JSON Object that contains specified field, within this node or its descendants. If no matching field is found in this node or its descendants, returns null.
Working with Tree Model Nodes in Jackson, This quick tutorial will show how to use Jackson 2 to convert a JSON String to a JsonNode (com.fasterxml.jackson.databind.JsonNode). public abstract JsonNode findParent(String fieldName) Method for finding a JSON Object that contains specified field, within this node or its descendants. If no matching field is found in this node or its descendants, returns null.
Typescript deserialize json
JSON to TypeScript class instance?, Just to be sure here's my question: What is actually the most robust and elegant automated solution for deserializing JSON to TypeScript runtime class instances The best solution I found when dealing with Typescript classes and json objects: add a constructor in your Typescript class that takes the json data as parameter. In that constructor you extend your json object with jQuery, like this: $.extend( this, jsonData). $.extend allows keeping the javascript prototypes while adding the json object's
How to parse JSON string in Typescript, Typescript is (a superset of) javascript, so you just use JSON.parse as you would in javascript: let obj = JSON.parse(jsonString);. Only that in typescript you can TypeScript: Working with JSON Sat, Mar 19, 2016. EDITS: Calling toString on Date is for illustrative purposes.; There’s a full commented example at the end. Use toJSON method as suggested by Schipperz.
json2typescript, Provides TypeScript methods to map a JSON object to a JavaScript object Tip: All serialize() and deserialize() methods may throw an Error in I'm using typescript for a project and need to serialize a collection to json, save it to a file and later deserialize that file into a similar collection. The collection looks something like: elements: Array<tool>
Jsonnode to string
Jackson JsonNode, The simplest and straightforward way to pretty print JsonNode is using the toPrettyString() method, as shown below: try { // JSON string String In this short tutorial, you'll learn how to parse a JSON string to a JsonNode object and vice versa using the Jackson library. Convert JSON String to JsonNode. To convert a JSON string to JsonNode, you can use the readTree() method from ObjectMapper. This method builds a tree model for all nodes and returns the root of the tree:
Pretty Print JsonNode to JSON String using Jackson, ObjectMapper mapper = new ObjectMapper(); JsonNode treeNode = mapper. readTree(parser); return treeNode. toString(); This will get you String containing the value of the 'data'. Filed under note to self as I keep forgetting this. Here’s a very simple way to pretty print a com.fasterxml.jackson.databind.JsonNode:
Jackson read value as string, public String prettyPrintJsonString(JsonNode jsonNode) { try { ObjectMapper mapper = new ObjectMapper(); Object json = mapper. It should be so simple, but I just cannot find it after being trying for an hour #embarrasing. I need to get a JSON string, for example, {'k1':v1,'k2':v2}, parsed as a JsonNode. JsonFactory facto
Json to typescript class
json2ts, generate TypeScript interfaces from JSON. email; feedback; help. generate TypeScript. © 2020 - Timmy Kokke. based on JSON C# Class Generator. generate TypeScript interfaces from json. json2ts. generate TypeScript interfaces from JSON based on JSON C# Class Generator
JSON to TypeScript • quicktype, Generate TypeScript interfaces with runtime validation code from JSON, JSON Schema, and GraphQL queries. Generate TypeScript NowInstall VSCode Assuming the json has the same properties as your typescript class, you don't have to copy your Json properties to your typescript object. You will just have to construct your Typescript object passing the json data in the constructor. In your ajax callback, you receive a company:
MakeTypes from JSON samples, MakeTypes generates TypeScript classes that parse and typecheck JSON objects at runtime, and let you statically type check code that interacts with JSON objects. All it requires is a set of JSON samples. Proxy classes generated with MakeTypes will parse your JSON and check that it matches the expected type at runtime. Statically type check code that interacts with JSON objects Proxy objects generated with MakeTypes are expressed as TypeScript classes, so you can statically type check that your code is appropriately accessing fields on the JSON
Typescript Deserialize Json To Class C
Create json object in 'typescript' dynamically
Taming Dynamic Data in TypeScript, Let's consider some JSON that we want to parse: For example, we might make a typo: To fully understand the quandary that we're in, it's helpful to look at how other static languages turn dynamic data into typed objects. If you are using Typescript, presumably you want to use the type safety; in which case naked Object and 'any' are counterindicated. Better to not use Object or {}, but some named type; or you might be using an API with specific types, which you need extend with your own fields.
How to create json schema object dynamically in typescript like this , I come to know that I again need to do json.parse after doing json.stringify. so using this line this.FormValueData The above JSON object can be sent by a server to a web page or any other client-side application. If we clearly observe, the structure of the JSON object is equivalent to that of the Typescript class, but what if we want to access the methods of the Todo class for the JSON object!
How to Cast a JSON Object Inside of TypeScript Class , We use JSON objects to store and transport data between a server and a client application Data 1: Suppose, we have a Typescript class defined in the client-side: declare module namespace { export interface RootObject { userId: number; How to Dynamically Add/Remove Table Rows using jQuery ? create object with dynamic keys in typescript RSS. 3 replies Last post Mar 26, 2018 03:16 AM by Brando ZWZ ‹ Previous Thread | Next Thread › Print Share
Es6 json to class
Serializing an ES6 class object as JSON, As with any other object you want to stringify in JS, you can use JSON.stringify : JSON.stringify(yourObject);. class MyClass { constructor() { this.foo = 3 } } var Serializing an ES6 class object as JSON. Ask Question Asked 3 years, 10 months ago. Active 6 months ago. -> json obj to class classToPlain() -> class to json obj
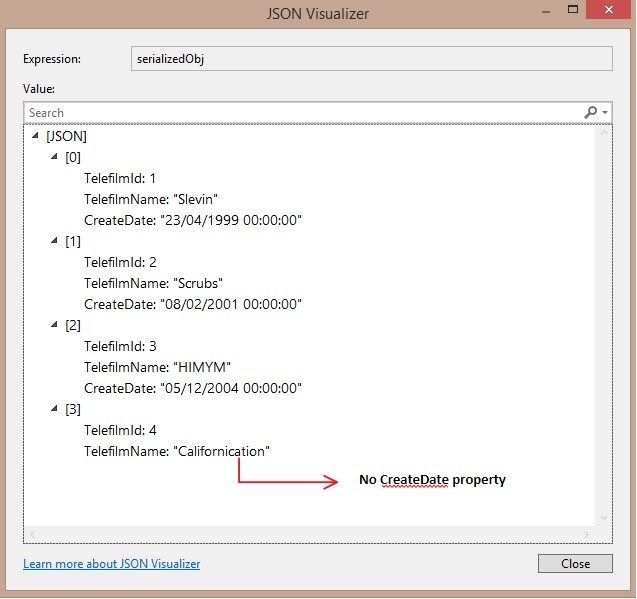
Mapping JSON to ES6 Classes, You might try using the reviver parameter of JSON.parse . Here is a simplified example: class Person { // Destructure the JSON object into the parameters with ECMAScript 2015. ES6, also known as ECMAScript2015, introduced classes. A class is a type of function, but instead of using the keyword function to initiate it, we use the keyword class, and the properties are assigned inside a constructor() method.
How to Convert a JavaScript / ES6 Object to a Class, load a json file via AJAX, filesystem, etc. return config. },. printConfigFormatInstructions() Configure package.json to run Babel. In order for our project to know that we want to run Babel, we need to specify a build script in package.json. In the package.json file, there is a property named scripts that holds an object for specifying command line shortcuts. We need to add a build property so it looks like this:
Jsonnode array
Typescript Deserialize Json To Class 8
How to convert JsonNode to ArrayNode using Jackson API in Java?, Is there a method equivalent to getJSONArray in org.json so that I have proper error handling in case it isn't an array? share. Yes, the Jackson manual parser design is quite different from other libraries. In particular, you will notice that JsonNode has most of the functions that you would typically associate with array nodes from other API's.
Jackson how to transform JsonNode to ArrayNode without casting , The answer is mostly in the Jackson documentation and the tutorial;. you have several ways to transform that JsonNode into useful data. A JsonNode is Jackson's tree model for JSON and it can read JSON into a JsonNode instance and write a JsonNode out to JSON. To read JSON into a JsonNode with Jackson by creating ObjectMapper instance and call the readValue() method. We can access a field, array or nested object using the get() method of JsonNode class.
Typescript Deserialize Json To Class Code
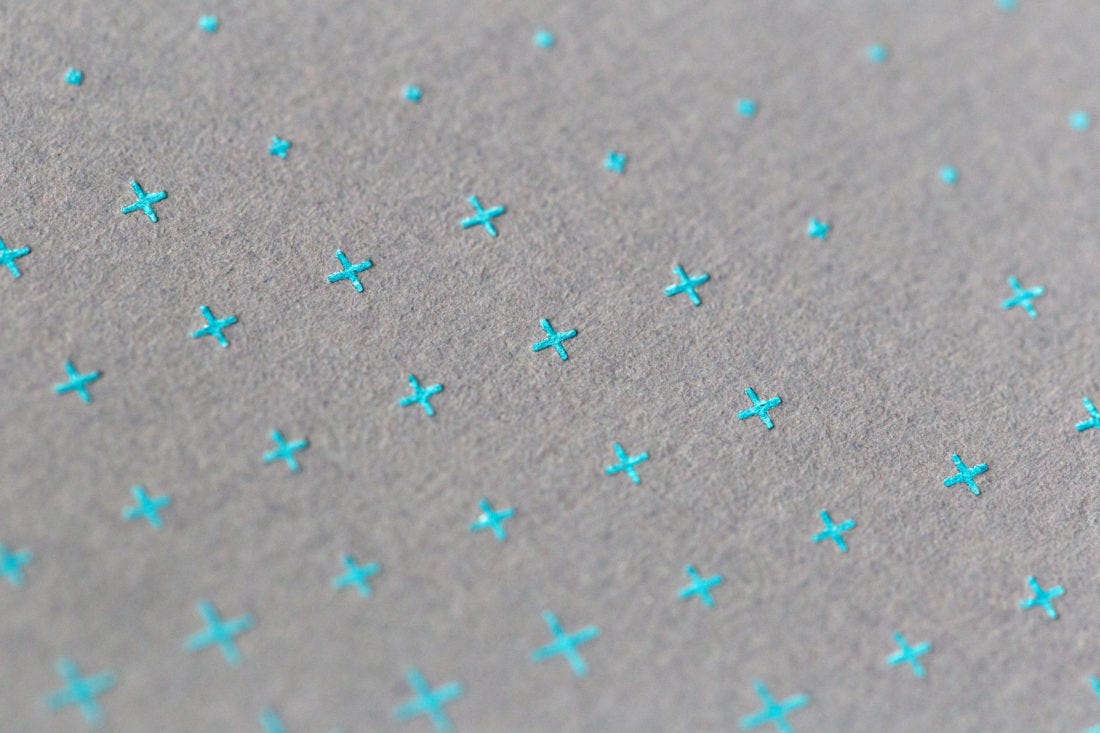
Converting JsonNode to java array, insert. public ArrayNode insert(int index, JsonNode value). Method for inserting specified child node as an element of this Array. If index is Method for accessing value of the specified element of an array node. For other nodes, null is always returned. For array nodes, index specifies exact location within array and allows for efficient iteration over child elements (underlying storage is guaranteed to be efficiently indexable, i.e. has random-access to elements).